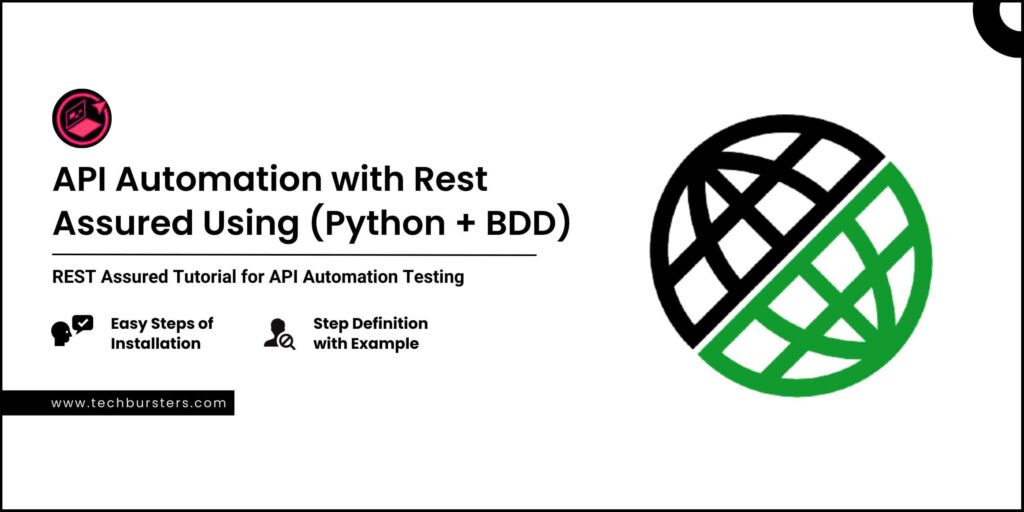
API Automation with Rest-Assured
In this blog, our experts share quick steps that will help you to automate API with Rest-Assured by use of Python + BDD.
Let’s start with the simple steps!
Step 1: Install Required Libraries
- Behave: Behave is the BDD (Behavior Driven Development) testing framework for Python. | pip install behave
- Requests: The requests library is commonly used for sending HTTP requests. | pip install requests
- Behave Rest API: This is an extension for Behave that provides support for testing REST APIs. | pip install behave-rest-API (For Installation)
- Jsonpath-Rw-Ext: Jsonpath-rw-ext is a library for extracting values from JSON responses using JSONPath expressions. | pip install jsonpath-rw-ext (For Installation)
Step 2: Write Feature File
- Create a feature file (e.g., features/API.feature) using Gherkin syntax:
Feature: API Testing with RestAssured
- Scenario: Verify GET Request
Given the API base URL is “https://smalltool.example.com”
When a GET request is made to “/endpoint”
Then the response status code expected 200
And the response should contain “expected_content”
# features/put_request.feature
- Feature: Update an existing resource using the API
- Implement Step Definitions
Create step definitions to link Gherkin with Python. For example, create a file named steps/api_steps.py:
Get Request
from behave import given, when, then
import requests
base_url = "https://api.example.com"
@given('the API endpoint is "{endpoint}"')
def step_given_api_endpoint(context, endpoint):
context.endpoint = f"{base_url}{endpoint}"
@when('a GET request is sent')
def step_when_get_request_sent(context):
context.response = requests.get(context.endpoint)
@then('the response status code should be {status_code:d}')
def step_then_check_status_code(context, status_code):
assert context.response.status_code == status_code, f"Expected {status_code}, but got {context.response.status_code}"
@then('the response should contain user details')
def step_then_check_user_details(context):
user_data = context.response.json()
assert "user_id" in user_data, "User ID not found in response"
Post Request
- Write Feature File
Write a feature file (e.g., features/api.feature) using Gherkin syntax:
Feature: API Testing with RestAssured
Scenario: Add a new user
Given the API endpoint is “/users”
When a POST request is sent with user data
Then the response status code expected 201
And the response should contain the new user’s details
- Implement Step Definitions
from behave import given, when, then
import requests
base_url = "https://api.example.com"
headers = {'Content-Type': 'application/json'}
@given('the API endpoint is "{endpoint}"')
def step_given_api_endpoint(context, endpoint):
context.endpoint = f"{base_url}{endpoint}"
@when('a POST request is sent with user data')
def step_when_post_request_sent(context):
user_data = {
"name": "John Doe",
"email": "john.doe@example.com",
"role": "user"
}
context.response = requests.post(context.endpoint, json=user_data, headers=headers)
@then('the response status code should be {status_code:d}')
def step_then_check_status_code(context, status_code):
assert context.response.status_code == status_code, f"Expected {status_code}, but got {context.response.status_code}"
@then('the response should contain the new user\'s details')
def step_then_check_new_user_details(context):
new_user_data = context.response.json()
assert "user_id" in new_user_data, "User ID not found in response"
assert new_user_data["name"] == "John Doe", "Incorrect user name in response"
assert new_user_data["email"] == "john.doe@example.com", "Incorrect email in response"
assert new_user_data["role"] == "user", "Incorrect user role in response"
Put Request
- Write Feature File
Write a feature file (e.g., features/api.feature) using Gherkin syntax:
Feature: API Testing with RestAssured
Scenario: Verify put request –
Update user information
Given the API endpoint is “/users/1”
When a PUT request is sent with updated user data
Then the response status code expected 200
And the response should confirm the user details have been updated
- Implement Step Definitions
Create step definitions to link Gherkin with Python. For example, create a file named steps/api_steps.py:
from behave import given, when, then
import requests
base_url = "https://api.example.com"
headers = {'Content-Type': 'application/json'}
@given('the API endpoint is "{endpoint}"')
def step_given_api_endpoint(context, endpoint):
context.endpoint = f"{base_url}{endpoint}"
@when('a PUT request is sent with updated user data')
def step_when_put_request_sent(context):
updated_user_data = {
"name": "Updated User",
"email": "updated.user@example.com",
"role": "admin"
}
context.response = requests.put(context.endpoint, json=updated_user_data, headers=headers)
@then('the response status code should be {status_code:d}')
def step_then_check_status_code(context, status_code):
assert context.response.status_code == status_code, f"Expected {status_code}, but got {context.response.status_code}"
@then('the response should confirm the user details have been updated')
def step_then_check_user_details_updated(context):
updated_user_data = context.response.json()
assert "user_id" in updated_user_data, "User ID not found in response"
assert updated_user_data["name"] == "Updated User", "Incorrect user name in response"
assert updated_user_data["email"] == "updated.user@example.com", "Incorrect email in response"
assert updated_user_data["role"] == "admin", "Incorrect user role in response"
Delete Request
- Write Feature File
Write a feature file (e.g., features/api.feature) using Gherkin syntax:
Feature: API Testing with RestAssured
Scenario: Delete user by ID
Given the API endpoint is “/users/1”
When a DELETE request is sent
Then the response status code expected 204
And the resource should be successfully deleted
- Implement Step Definitions
Create step definitions to link Gherkin with Python. For example, create a file named steps/api_steps.py:
from behave import given, when, then
import requests
base_url = "https://api.example.com"
@given('the API endpoint is "{endpoint}"')
def step_given_api_endpoint(context, endpoint):
context.endpoint = f"{base_url}{endpoint}"
@when('a DELETE request is sent')
def step_when_delete_request_sent(context):
context.response = requests.delete(context.endpoint)
@then('the response status code should be {status_code:d}')
def step_then_check_status_code(context, status_code):
assert context.response.status_code == status_code, f"Expected {status_code}, but got {context.response.status_code}"
@then('the resource should be successfully dele0ted')
def step_then_check_resource_deleted(context):
# Additional checks can be added to verify that the resource has been deleted
assert context.response.status_code == 204, "Expected a 204 status code for successful deletion"
Related Post:-